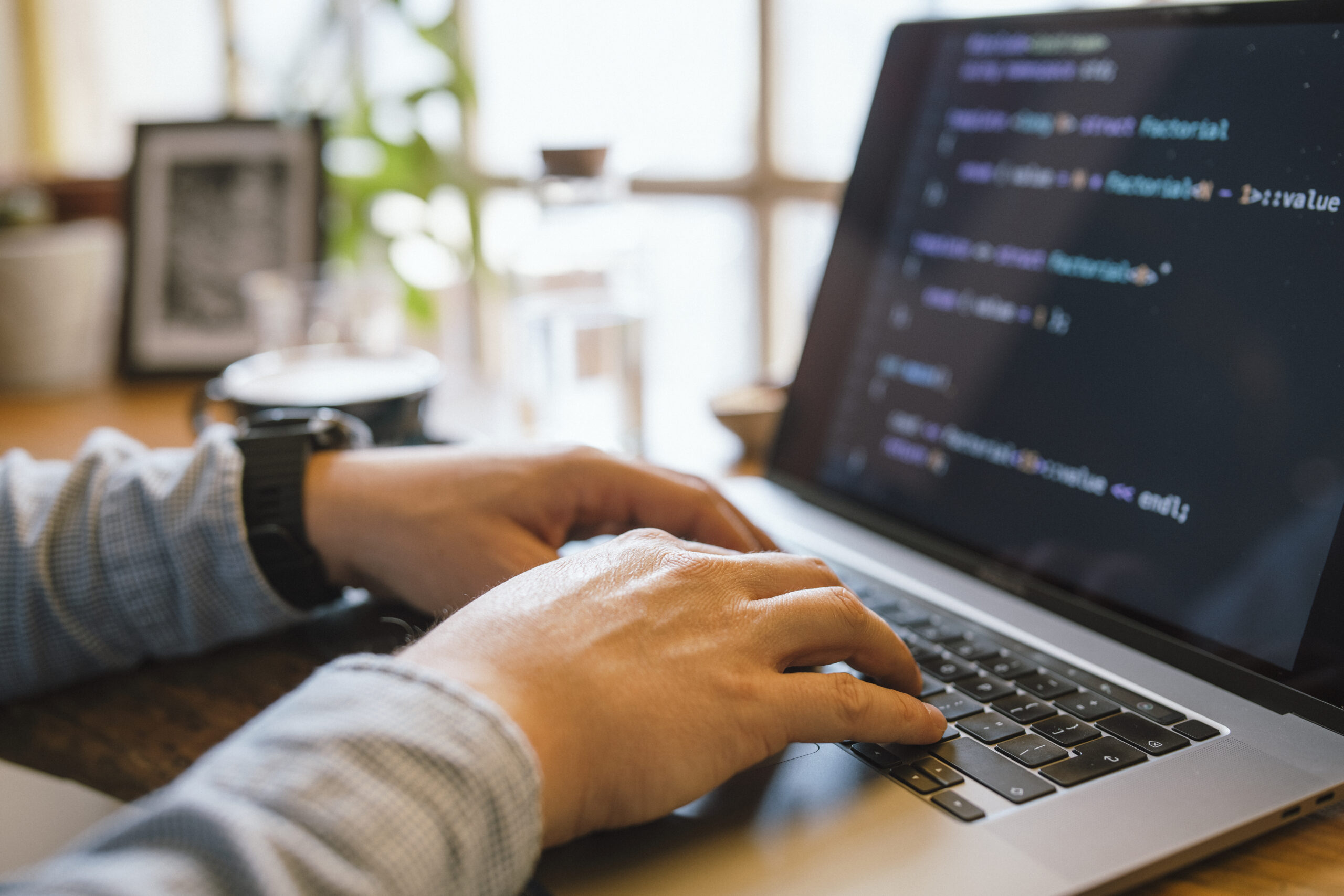
Debugging is Just about the most necessary — yet usually neglected — competencies in a developer’s toolkit. It isn't nearly fixing broken code; it’s about comprehension how and why factors go Erroneous, and Discovering to Imagine methodically to unravel complications competently. Whether or not you're a beginner or perhaps a seasoned developer, sharpening your debugging abilities can conserve hours of frustration and dramatically help your efficiency. Here's various tactics that will help developers level up their debugging activity by me, Gustavo Woltmann.
Master Your Tools
Among the quickest means builders can elevate their debugging techniques is by mastering the instruments they use every single day. Although composing code is a single Portion of improvement, understanding how to connect with it proficiently for the duration of execution is equally important. Modern-day progress environments arrive equipped with powerful debugging abilities — but several developers only scratch the area of what these tools can perform.
Acquire, by way of example, an Integrated Improvement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to established breakpoints, inspect the value of variables at runtime, step through code line by line, and perhaps modify code to the fly. When utilised appropriately, they let you observe specifically how your code behaves all through execution, and that is a must have for monitoring down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for entrance-conclusion developers. They enable you to inspect the DOM, keep track of network requests, look at authentic-time effectiveness metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can switch annoying UI issues into manageable duties.
For backend or method-stage developers, resources like GDB (GNU Debugger), Valgrind, or LLDB offer deep control above operating processes and memory administration. Learning these tools may have a steeper Mastering curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Past your IDE or debugger, turn out to be snug with Variation Regulate units like Git to know code background, come across the precise second bugs had been launched, and isolate problematic improvements.
Ultimately, mastering your equipment suggests going over and above default options and shortcuts — it’s about developing an intimate familiarity with your enhancement atmosphere to ensure when concerns occur, you’re not dropped in the dead of night. The better you realize your equipment, the greater time you'll be able to spend fixing the actual trouble in lieu of fumbling as a result of the method.
Reproduce the Problem
One of the more vital — and infrequently ignored — steps in efficient debugging is reproducing the issue. In advance of jumping into your code or producing guesses, developers require to create a reliable ecosystem or circumstance exactly where the bug reliably appears. Without reproducibility, fixing a bug will become a activity of probability, usually leading to wasted time and fragile code alterations.
The initial step in reproducing an issue is collecting as much context as feasible. Talk to issues like: What actions led to the issue? Which natural environment was it in — improvement, staging, or generation? Are there any logs, screenshots, or mistake messages? The greater depth you have, the simpler it becomes to isolate the precise ailments beneath which the bug takes place.
When you’ve collected adequate facts, endeavor to recreate the trouble in your neighborhood ecosystem. This could signify inputting precisely the same details, simulating comparable consumer interactions, or mimicking method states. If the issue appears intermittently, consider creating automated checks that replicate the edge circumstances or condition transitions concerned. These exams not only assistance expose the trouble but also avoid regressions in the future.
Often, The difficulty could be ecosystem-precise — it'd come about only on selected functioning systems, browsers, or under unique configurations. Making use of resources like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms is usually instrumental in replicating these kinds of bugs.
Reproducing the issue isn’t simply a stage — it’s a mindset. It calls for endurance, observation, and a methodical method. But when you finally can continuously recreate the bug, you're presently midway to fixing it. With a reproducible circumstance, you can use your debugging resources far more proficiently, exam potential fixes safely, and communicate a lot more Obviously using your staff or end users. It turns an summary grievance into a concrete problem — and that’s in which developers thrive.
Read and Understand the Mistake Messages
Mistake messages are sometimes the most useful clues a developer has when anything goes Mistaken. As an alternative to viewing them as irritating interruptions, developers should really master to take care of error messages as direct communications from the program. They typically inform you just what happened, where it transpired, and occasionally even why it happened — if you know how to interpret them.
Start by examining the information very carefully and in entire. Several developers, particularly when below time force, glance at the first line and promptly start off creating assumptions. But further inside the error stack or logs may well lie the correct root cause. Don’t just duplicate and paste mistake messages into search engines like google and yahoo — read through and comprehend them initially.
Break the mistake down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it stage to a selected file and line quantity? What module or functionality induced it? These thoughts can information your investigation and issue you toward the liable code.
It’s also valuable to understand the terminology on the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java normally stick to predictable styles, and Understanding to acknowledge these can considerably quicken your debugging course of action.
Some problems are vague or generic, As well as in Those people circumstances, it’s important to examine the context where the mistake occurred. Check out associated log entries, input values, and up to date adjustments during the codebase.
Don’t forget about compiler or linter warnings possibly. These frequently precede more substantial issues and provide hints about probable bugs.
Ultimately, error messages are usually not your enemies—they’re your guides. Finding out to interpret them correctly turns chaos into clarity, aiding you pinpoint difficulties faster, decrease debugging time, and become a additional economical and confident developer.
Use Logging Wisely
Logging is The most highly effective tools inside a developer’s debugging toolkit. When used efficiently, it provides true-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood without needing to pause execution or step through the code line by line.
An excellent logging approach starts off with knowing what to log and at what level. Prevalent logging concentrations involve DEBUG, INFO, WARN, ERROR, and FATAL. Use DEBUG for comprehensive diagnostic details in the course of improvement, INFO for general events (like effective start-ups), Alert for opportunity difficulties that don’t crack the appliance, ERROR for real problems, and Lethal if the process can’t continue.
Stay clear of flooding your logs with too much or irrelevant knowledge. Excessive logging can obscure crucial messages and slow down your procedure. Center on crucial events, point out improvements, input/output values, and critical final decision factors in the code.
Format your log messages clearly and continuously. Incorporate context, including timestamps, ask for IDs, and function names, so it’s much easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all with out halting This system. They’re Specially beneficial in generation environments exactly where stepping by code isn’t achievable.
On top of that, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. That has a perfectly-believed-out logging solution, you'll be able to lessen the time it takes to spot difficulties, acquire deeper visibility into your purposes, and improve the Total maintainability and trustworthiness of one's code.
Consider Similar to a Detective
Debugging is not just a complex endeavor—it is a type of investigation. To correctly determine and correct bugs, builders should tactic the process similar to a detective resolving a thriller. This mindset can help stop working intricate difficulties into workable parts and adhere to clues logically to uncover the basis bring about.
Get started by gathering evidence. Consider the symptoms of the challenge: mistake messages, incorrect output, or efficiency difficulties. Identical to a detective surveys a crime scene, collect as much appropriate facts as you could with out jumping to conclusions. Use logs, check conditions, and person stories to piece alongside one another a transparent picture of what’s going on.
Subsequent, form hypotheses. Check with you: What could possibly be producing this conduct? Have any modifications a short while ago been designed towards the codebase? Has this challenge happened before under similar conditions? The goal is usually to slender down choices and determine prospective culprits.
Then, test your theories systematically. Seek to recreate the condition in a very managed setting. Should you suspect a specific functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, request your code questions and Permit the outcome lead you nearer to the truth.
Pay out shut awareness to modest specifics. Bugs often disguise while in the least envisioned areas—like a lacking semicolon, an off-by-one particular mistake, or perhaps a race ailment. Be comprehensive and patient, resisting the urge to patch The difficulty without having absolutely knowledge it. Short term fixes might disguise the true issue, just for it to resurface later on.
Lastly, continue to keep notes on Whatever you tried using and acquired. Just as detectives log their investigations, documenting your debugging system can help save time for foreseeable future troubles and aid others have an understanding of your reasoning.
By pondering similar to a detective, developers can sharpen their analytical capabilities, technique challenges methodically, and grow to be simpler at uncovering concealed concerns in elaborate techniques.
Compose Tests
Crafting assessments is among the best tips on how to help your debugging abilities and General growth efficiency. Assessments not simply help catch bugs early but also serve as a safety net that provides you self-confidence when earning variations in your codebase. A nicely-analyzed software is much easier to debug since it allows you to pinpoint just where and when an issue happens.
Get started with unit assessments, which target person capabilities or modules. These modest, isolated tests can speedily reveal no matter if a selected piece of logic is Performing as anticipated. Any time a examination fails, you instantly know in which to search, significantly decreasing the time spent debugging. Unit checks are Primarily useful for catching regression bugs—issues that reappear after previously getting set.
Subsequent, combine integration assessments and conclude-to-stop tests into your workflow. These help ensure that several areas of your software perform together effortlessly. They’re specially beneficial for catching bugs that happen in elaborate programs with several factors or expert services interacting. If one thing breaks, your tests can inform you which Portion of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Consider critically about your code. To check a function thoroughly, you may need to know its inputs, predicted outputs, and edge instances. This standard of comprehending The natural way prospects to raised code structure and less bugs.
When debugging an issue, composing a failing exam that reproduces the bug is usually a strong starting point. Once the examination fails consistently, it is possible to deal with fixing the bug and look at your test move when The difficulty is resolved. This strategy makes sure that the same bug doesn’t return Sooner or later.
In short, composing checks turns debugging from more info a annoying guessing activity into a structured and predictable procedure—supporting you capture extra bugs, quicker and a lot more reliably.
Choose Breaks
When debugging a tough problem, it’s effortless to be immersed in the problem—looking at your display screen for hrs, seeking solution right after Resolution. But One of the more underrated debugging tools is simply stepping away. Taking breaks assists you reset your brain, lessen annoyance, and infrequently see The problem from the new point of view.
When you are way too near the code for far too extensive, cognitive exhaustion sets in. You may perhaps get started overlooking obvious errors or misreading code which you wrote just hours earlier. Within this state, your Mind results in being fewer economical at trouble-resolving. A brief stroll, a coffee break, or simply switching to another undertaking for ten–15 minutes can refresh your concentrate. Numerous developers report finding the foundation of a challenge once they've taken time for you to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could out of the blue observe a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
If you’re trapped, an excellent rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 minute split. Use that point to maneuver all around, stretch, or do anything unrelated to code. It could sense counterintuitive, In particular less than limited deadlines, nonetheless it really leads to faster and more practical debugging Over time.
To put it briefly, getting breaks just isn't an indication of weak spot—it’s a smart approach. It provides your Mind Room to breathe, improves your point of view, and will help you stay away from the tunnel vision That always blocks your progress. Debugging is a psychological puzzle, and rest is part of resolving it.
Learn From Just about every Bug
Every single bug you experience is a lot more than just A brief setback—It really is an opportunity to develop for a developer. No matter if it’s a syntax error, a logic flaw, or possibly a deep architectural problem, each one can teach you a thing valuable in case you make the effort to mirror and analyze what went Improper.
Get started by inquiring yourself several essential questions after the bug is settled: What brought about it? Why did it go unnoticed? Could it are caught earlier with much better techniques like device tests, code evaluations, or logging? The solutions generally reveal blind spots with your workflow or understanding and assist you to Construct more powerful coding behaviors transferring ahead.
Documenting bugs can even be a wonderful practice. Retain a developer journal or retain a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After some time, you’ll begin to see patterns—recurring problems or typical mistakes—that you can proactively avoid.
In team environments, sharing Anything you've figured out from a bug together with your peers is usually In particular highly effective. No matter if it’s by way of a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other folks avoid the exact challenge boosts crew efficiency and cultivates a much better Understanding culture.
Extra importantly, viewing bugs as lessons shifts your state of mind from irritation to curiosity. In place of dreading bugs, you’ll commence appreciating them as important aspects of your growth journey. In the end, many of the greatest builders are certainly not the ones who produce ideal code, but individuals that constantly master from their blunders.
Eventually, Each and every bug you take care of adds a completely new layer for your ability established. So up coming time you squash a bug, have a moment to mirror—you’ll occur away a smarter, extra capable developer thanks to it.
Conclusion
Increasing your debugging expertise usually takes time, practice, and endurance — though the payoff is big. It makes you a more successful, self-assured, and capable developer. The next time you are knee-deep in a very mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to be much better at Anything you do.